Getting Started
Installation
For now, Virdant only works on Linux.
You can install it by cloning the repository and running make install
:
$ git clone https://github.com/virdant-lang/virdant
$ cd virdant
$ make install
This will build Virdant from source and then copies the binaries to $HOME/.local/virdant/bin
.
Make sure that this directory is on your $PATH
.
Blinky
Tradition dictates that the first program that is written in any programming language should be Hello, World! In that same spirit, here is a small design which blinks an LED on and off:
1mod Top {
2 incoming clock : Clock;
3 outgoing led : Bit;
4
5 reg led_on : Bit on clock;
6 led_on <= led_on->not();
7
8 led := led_on;
9}
Compiling to Verilog
We can compile this design to Verilog with the following:
$ vir compile blink.vir
The result will be a new file called build/blink.v
.
To simulate the design, you need a Verilog testbench. Here is one which will run the design for 100 cycles:
1module Testbench();
2 initial begin
3 $dumpfile("build/out.vcd");
4 $dumpvars(0, top);
5 end
6
7 reg clock = 1'b0;
8 always #(5) clock = !clock;
9
10 Top top(
11 .clock(clock)
12 );
13
14 reg [31:0] cycles = 100;
15
16 always @(posedge clock) begin
17 cycles <= cycles - 1;
18 if (cycles == 0) begin
19 $finish;
20 end
21 end
22endmodule
If you have Icarus Verilog installed, you can compile a simulator and run it with this with these commands:
$ iverilog testbench.v build/blink.v -o build/blink
$ ./build/blink
VCD info: dumpfile build/out.vcd opened for output.
Finally, using a waveform viewer such as GTK Wave, you can view the waveform:
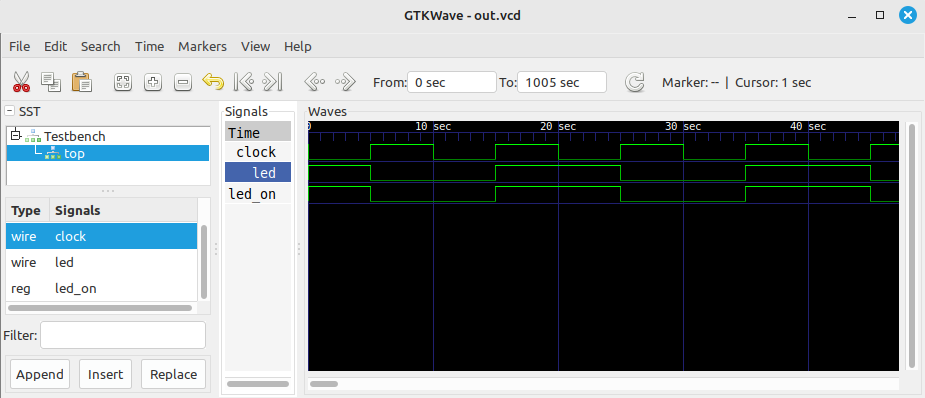